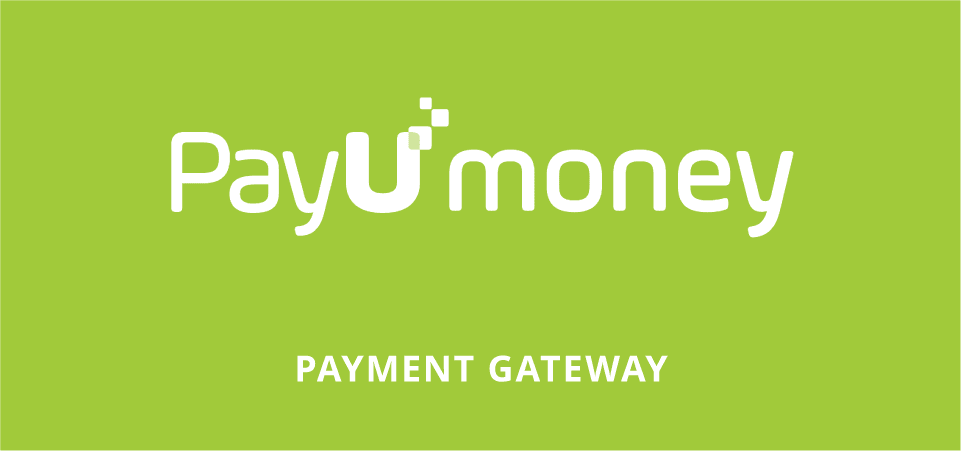
Introduction
PayU Money is a popular payment gateway that allows businesses to accept online payments seamlessly. In this guide, we will demonstrate how to create a payment link using PayU Money in a Laravel application.
Prerequisites
Before proceeding, ensure you have the following:
- A Laravel project set up
- PayU Money account with API credentials
- Composer installed.
Step 1: Define the Route
In routes/web.php
, add the following route to handle payment link generation:
use App\Http\Controllers\PayUController;
use Illuminate\Support\Facades\Route;
Route::post('/payu/payment-link', [PayUController::class, 'generatePaymentLink']);
This route is responsible for processing the payment link request.
Step 2: Create the PayU Controller
Inside app/Http/Controllers/PayUController.php
, create the PayUController
with the following code:
namespace App\Http\Controllers;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Log;
use GuzzleHttp\Client;
class PayUController extends Controller
{
public function generatePaymentLink(Request $request)
{
$token = $this->getAuthToken();
if (!$token) {
Log::error('PayU Auth Token missing');
return response()->json(['success' => false, 'error' => 'Auth token missing'], 500);
}
try {
$payload = json_encode([
"source" => "API",
"subAmount" => $request->amount, // Payment Link Amount.
"currency" => "INR",
"description" => $request->description, // Payment Link Title.
"customer" => [
"name" => $request->name, // Payment Client Name.
"email" => $request->email, // Payment Client Email.
"phone" => $request->phone // Payment Client Phone Number.
]
]);
$response = (new Client())->post("https://oneapi.payu.in/payment-links", [
'headers' => ['Authorization' => "Bearer $token",
'Content-Type' => 'application/json',
'merchantId' => 'YOUR_MERCHANT_ID'],
'body' => $payload]);
$responseData = json_decode($response->getBody(), true);
return response()->json([
'success' => true,
'payment_url' => $responseData['result']['paymentLink'] ?? '',
'payment_data' => $responseData
]);
} catch (\Exception $e) {
return response()->json(['success' => false, 'error' => $e->getMessage()], 500);
}
}
public function getAuthToken()
{
try {
$response = (new Client())->post("https://accounts.payu.in/oauth/token", [
'headers' => ['accept' => 'application/json',
'content-type' => 'application/x-www-form-urlencoded'],
'form_params' => [
'grant_type' => 'client_credentials',
'client_id' => "YOUR_CLIENT_ID",
'client_secret' => "YOUR_CLIENT_SECRET",
'scope' => 'create_payment_links',
]]);
$data = json_decode($response->getBody(), true);
if (empty($data['access_token'])) throw new \Exception('Access token not received');
return $data['access_token'];
} catch (\Exception $e) {
return response()->json(['success' => false, 'error' => $e->getMessage()], 500);
}
}
}
Replace YOUR_CLIENT_ID
, YOUR_CLIENT_SECRET
, and YOUR_MERCHANT_ID
with your actual PayU credentials.
Step 3: Create the Blade Template
In your resources/views/
directory, create a Blade template for the payment form.
<form action="{{ url('/payu/payment-link') }}" method="POST">
@csrf
<label for="amount">Amount:</label>
<input type="text" id="amount" name="amount" required>
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<label for="phone">Phone:</label>
<input type="text" id="phone" name="phone" required>
<label for="description">Description:</label>
<input type="text" id="description" name="description" required>
<button type="submit">Generate Payment Link</button>
</form>
Step 4: Testing the Payment Link Generation
To test the payment link generation:
- Start your Laravel server:
php artisan serve
- Navigate to the form URL.
- Fill in the details and submit the form.
- The system should generate a PayU payment link and return it in JSON format.
Sample Response
{
"status": 0,
"message": "paymentLink generated",
"result": {
"subAmount": 2,
"tax": 0,
"shippingCharge": 0,
"totalAmount": 2,
"invoiceNumber": "INV7711514022032",
"paymentLink": "http://pp72.pmny.in/MIioqucT8hXV",
"description": "paymentLink for testing",
"active": true,
"isPartialPaymentAllowed": false,
"expiryDate": "2023-03-21 17:58:30",
"udf": {
"udf1": null,
"udf2": null,
"udf3": null,
"udf4": null,
"udf5": null
},
"address": {
"line1": null,
"line2": null,
"city": null,
"state": null,
"country": null,
"zipCode": null
},
"emailStatus": "not opted",
"smsStatus": "not opted"
},
"errorCode": null,
"guid": null
}
Conclusion
You have successfully integrated PayU Money’s payment link generation feature in your Laravel application. You can further enhance this by storing transaction details, sending automated notifications, and handling webhook responses for successful or failed payments.
Happy coding!